In the standard request handler usage scenarios, there is always a complete URL, which is send to the server. Then the server processes that and returns the result page to the client.
With a little change, these standard request handlers in a module can be modified to be used from clients asynchronously by the usage of AJAX.
Client Part
The easiest approach is to use AJAX with JQUERY (which is delivered by the content server). If the base page was built from another request handler, it can include weblingo constructs. These are OSCRIPT parts, delimited by `-Delimiters. Like JSP, the final renderer evaluates everything between two delimiters and replaces that with the result of the evaluation.
So a AJAX JQUERY command with intermixed OSCRIPT parts can look like that:
This is a simple AJAX call, calling the request handler on the server as usual. But there is a parameter called func, which contains the actual request handler address and one parameter nodeid, which is the data parameter.
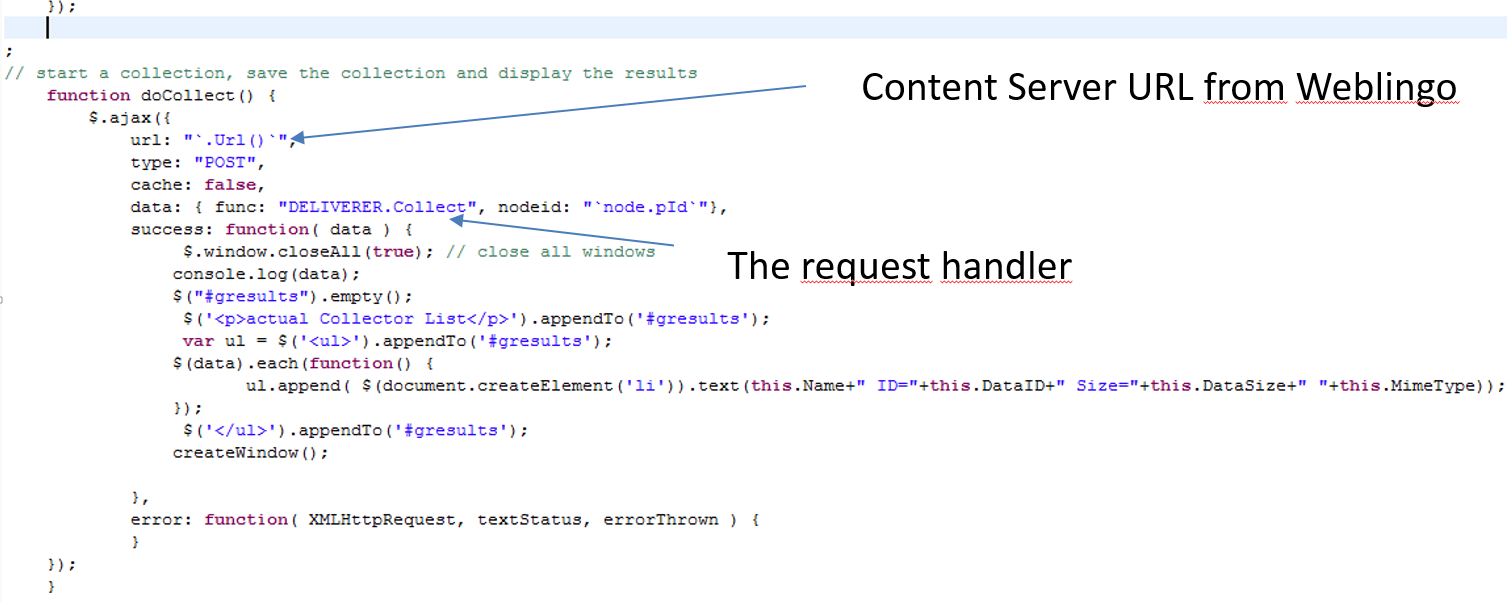
Server Part
A sample request handler looks like this listing below. There is a prototype declared, this prototype must exist in the request.
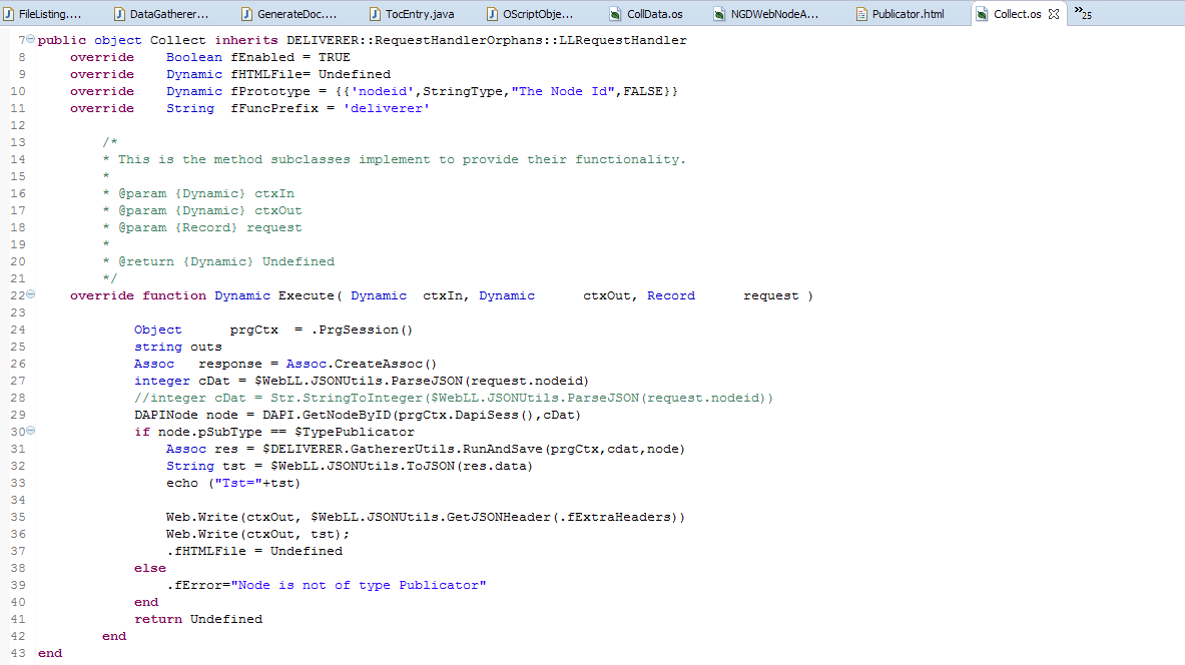
The rest of the data is encapsulated in the JSON array. This array can be parsed into an assoc with the call to
$WebLL.JSONUtils.ParseJSON(request.configData)
and processed in the standard way.
Any responses should be handled in the standard way with an assoc.
String tst = $WebLL.JSONUtils.ToJSON(response) Web.Write(ctxOut, $WebLL.JSONUtils.GetJSONHeader(.fExtraHeaders)) Web.Write(ctxOut, tst); .fHTMLFile = Undefined
This assoc is changed with a call to the ToJSON to a normal string.
Here, we do not have a output html file, so we have to use a Web.Write to write the output directly to the port. Do not forget to include the JSONHeaders.
The fHTMLFile stores normally the weblingo output file and has to be set to undefined in this case.
package DELIVERER::PUBLICATOR::AJAXHANDLER public object UpdateAjax inherits DELIVERER::RequestHandlerOrphans::LLRequestHandler override Boolean fEnabled = TRUE override String fFuncPrefix = 'deliverer' override Dynamic fHTMLFile override List fPrototype = { { 'configdata', -1, 'The config data changed at the client', FALSE } } /* * This is the Request handler to handle the Ajax Update Request(s) * Based on the original Request handlers, this handler receives an AJAX Request from the NodeAction Browse Page * and sends the Response back to the Browse Page (PUBLICATOR.html) * * @param {Dynamic} ctxIn * @param {Dynamic} ctxOut * @param {Record} request * * @return {Dynamic} Undefined */ override function Dynamic Execute( \ Dynamic ctxIn, \ Dynamic ctxOut, \ Record request ) Object prgCtx = .PrgSession() Assoc response = Assoc.CreateAssoc() Assoc cDat = $WebLL.JSONUtils.ParseJSON(request.configData) integer nodeid = cDat.id DAPINode node = DAPI.GetNodeByID(prgCtx.DapiSess(),nodeid) Assoc.Delete(cDat,"id") // remove the NodeID string remark = cDat.remark Assoc.Delete(cDat,"remark"); node.pComment=remark // save configdata in pExtendedData // do not forget to include other assocs, if needed from the pExtendedData Assoc a = node.pExtendedData; //TODO Add Things a.configdata=cDat node.pExtendedData = a DAPI.UpdateNode(node) // store it // Send response response.ok="OK" response.msg="Fine" String tst = $WebLL.JSONUtils.ToJSON(response) Web.Write(ctxOut, $WebLL.JSONUtils.GetJSONHeader(.fExtraHeaders)) Web.Write(ctxOut, tst); .fHTMLFile = Undefined return Undefined end end